React hooks for accessing the localStorage Web Storage API.
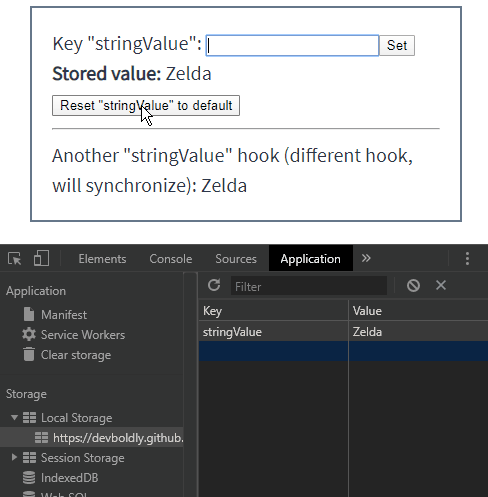
๐๏ธ Live Demo
For sessionStorage, see react-use-window-sessionstorage.
Overview
A set of hooks to easily store and retrieve data from localStorage.
Encoding is handled for common data types, including booleans, numbers, strings, and objects, or you can encode data yourself if you'd like.
Changes to localStorage are synchronized across all hooks automatically.
What is localStorage? The
localStorage
property allows you to store{key: value}
string data that is saved across browser sessions. Data stored inlocalStorage
has no expiration time.
For sessionStorage, check out the companion project react-use-window-sessionstorage.
Features include:
- ๐ช Easily add
localStorage
support- Store data across browser sessions with ease.
- ๐ข Support for primitives and objects
- Store and retrieve strings, booleans, numbers, and objects effortlessly.
- ๐ Default values
- Optional support for defaults is baked right in.
- ๐ Automatic synchronization
- Changes are synchronized across hooks automatically.
- ๐พ Customizable
- Want to store something unusual? Just provide your own encoder.
- โ Storage availability detection
- Detects if
localStorage
is available for use and lets you know otherwise.
- Detects if
- ๐งผ Clearing support
- Clear all localStorage values and reset hooks to defaults with one simple call.
Installation
npm i react-use-window-localstorage
Quick Start
Storing Strings
Use the useLocalStorageString hook:
import { useLocalStorageString } from "react-use-window-localstorage";
In your function component:
const defaultValue = "cyan";const [value, setValue] = useLocalStorageString("favColor", defaultValue);
Storing Objects
Use the useLocalStorageObject hook:
import { useLocalStorageObject } from "react-use-window-localstorage";
In your function component:
const defaultValue = { a: "hello", b: 123 };const [value, setValue] = useLocalStorageObject("myObj", defaultValue);
Note that your objects must be compatible with JSON.stringify(). Use useLocalStorageItem otherwise.
Storing Booleans
Use the useLocalStorageBoolean hook:
import { useLocalStorageBoolean } from "react-use-window-localstorage";
In your function component:
const defaultValue = true;const [value, setValue] = useLocalStorageBoolean("swordEquipped", defaultValue);
Storing Numbers
Use the useLocalStorageNumber hook:
import { useLocalStorageNumber } from "react-use-window-localstorage";
In your function component:
const defaultValue = 3.14159;const [value, setValue] = useLocalStorageNumber("importantNumber",defaultValue);
Note: All value defaults are optional. Hooks will return
null
if none is provided.
Storing Everything Else
If you'd like to store something other than the data types above, define your own encoding using the useLocalStorageItem hook.
Here's a starting point:
import { useLocalStorageItem } from "react-use-window-localstorage";
In your function component:
const defaultValue = "something custom";const encode = (value) => JSON.stringify(value);const decode = (itemString) => JSON.parse(itemString);const [value, setValue] = useLocalStorageItem("name",defaultValue,encode,decode);
Provide null
for no default value.
Additional Features
All hooks provide additional features in their return arrays, should you be interested:
const [value, setValue, loading, available, reset] = useLocalStorageString("favColor","cyan");
- A
loading
value oftrue
indicates that the value is being loaded from localStorage. - An
available
value oftrue
indicates thatlocalStorage
is supported and available for use. - The
reset()
function sets the value back to the provided default, ornull
if none was given.
Clearing localStorage
import { useClearLocalStorage } from "react-use-window-localstorage";
const clearLocalStorage = useClearLocalStorage();
Call clearLocalStorage()
to clear all values in localStorage using localStorage.clear()
and reset all hooks to their defaults (or null
if none provided).
TypeScript
Type definitions have been included for TypeScript support.
Contributing
Open source software is awesome and so are you. ๐
Feel free to submit a pull request for bugs or additions, and make sure to update tests as appropriate. If you find a mistake in the docs, send a PR! Even the smallest changes help.
For major changes, open an issue first to discuss what you'd like to change.
See Kindling for npm script documentation.
โญ Found It Helpful? Star It!
If you found this project helpful, let the community know by giving it a star: ๐โญ